勇哥来测试一下全部的查询运算符。
每天测试一部分,代码会持续更新中……
using System; using System.Collections; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace ConsoleApp1 { class Person { public string Name { set; get; } public int Age { set; get; } public override string ToString() { return $"{Name},{Age}"; } } class Department { public string Name { set; get; } public Person Employee { set; get; } public override string ToString() { return $"{Name}"; } } class Program { private static List<Racer> racers; public static IList<Racer> GetChampions() { if (racers == null) { racers = new List<Racer>(40); racers.Add(new Racer("Nino", "Farina", "Italy", 33, 5, new int[] { 1950 }, new string[] { "Alfa Romeo" })); racers.Add(new Racer("Alberto", "Ascari", "Italy", 32, 10, new int[] { 1952, 1953 }, new string[] { "Ferrari" })); racers.Add(new Racer("Juan Manuel", "Fangio", "Argentina", 51, 24, new int[] { 1951, 1954, 1955, 1956, 1957 }, new string[] { "Alfa Romeo", "Maserati", "Mercedes", "Ferrari" })); racers.Add(new Racer("Mike", "Hawthorn", "UK", 45, 3, new int[] { 1958 }, new string[] { "Ferrari" })); racers.Add(new Racer("Phil", "Hill", "USA", 48, 3, new int[] { 1961 }, new string[] { "Ferrari" })); racers.Add(new Racer("John", "Surtees", "UK", 111, 6, new int[] { 1964 }, new string[] { "Ferrari" })); racers.Add(new Racer("Jim", "Clark", "UK", 72, 25, new int[] { 1963, 1965 }, new string[] { "Lotus" })); racers.Add(new Racer("Jack", "Brabham", "Australia", 125, 14, new int[] { 1959, 1960, 1966 }, new string[] { "Cooper", "Brabham" })); racers.Add(new Racer("Denny", "Hulme", "New Zealand", 112, 8, new int[] { 1967 }, new string[] { "Brabham" })); racers.Add(new Racer("Graham", "Hill", "UK", 176, 14, new int[] { 1962, 1968 }, new string[] { "BRM", "Lotus" })); racers.Add(new Racer("Jochen", "Rindt", "Austria", 60, 6, new int[] { 1970 }, new string[] { "Lotus" })); racers.Add(new Racer("Jackie", "Stewart", "UK", 99, 27, new int[] { 1969, 1971, 1973 }, new string[] { "Matra", "Tyrrell" })); racers.Add(new Racer("Emerson", "Fittipaldi", "Brazil", 143, 14, new int[] { 1972, 1974 }, new string[] { "Lotus", "McLaren" })); racers.Add(new Racer("James", "Hunt", "UK", 91, 10, new int[] { 1976 }, new string[] { "McLaren" })); racers.Add(new Racer("Mario", "Andretti", "USA", 128, 12, new int[] { 1978 }, new string[] { "Lotus" })); racers.Add(new Racer("Jody", "Scheckter", "South Africa", 112, 10, new int[] { 1979 }, new string[] { "Ferrari" })); racers.Add(new Racer("Alan", "Jones", "Australia", 115, 12, new int[] { 1980 }, new string[] { "Williams" })); racers.Add(new Racer("Keke", "Rosberg", "Finland", 114, 5, new int[] { 1982 }, new string[] { "Williams" })); racers.Add(new Racer("Niki", "Lauda", "Austria", 173, 25, new int[] { 1975, 1977, 1984 }, new string[] { "Ferrari", "McLaren" })); racers.Add(new Racer("Nelson", "Piquet", "Brazil", 204, 23, new int[] { 1981, 1983, 1987 }, new string[] { "Brabham", "Williams" })); racers.Add(new Racer("Ayrton", "Senna", "Brazil", 161, 41, new int[] { 1988, 1990, 1991 }, new string[] { "McLaren" })); racers.Add(new Racer("Nigel", "Mansell", "UK", 187, 31, new int[] { 1992 }, new string[] { "Williams" })); racers.Add(new Racer("Alain", "Prost", "France", 197, 51, new int[] { 1985, 1986, 1989, 1993 }, new string[] { "McLaren", "Williams" })); racers.Add(new Racer("Damon", "Hill", "UK", 114, 22, new int[] { 1996 }, new string[] { "Williams" })); racers.Add(new Racer("Jacques", "Villeneuve", "Canada", 165, 11, new int[] { 1997 }, new string[] { "Williams" })); racers.Add(new Racer("Mika", "Hakkinen", "Finland", 160, 20, new int[] { 1998, 1999 }, new string[] { "McLaren" })); racers.Add(new Racer("Michael", "Schumacher", "Germany", 287, 91, new int[] { 1994, 1995, 2000, 2001, 2002, 2003, 2004 }, new string[] { "Benetton", "Ferrari" })); racers.Add(new Racer("Fernando", "Alonso", "Spain", 177, 27, new int[] { 2005, 2006 }, new string[] { "Renault" })); racers.Add(new Racer("Kimi", "Räikkönen", "Finland", 148, 17, new int[] { 2007 }, new string[] { "Ferrari" })); racers.Add(new Racer("Lewis", "Hamilton", "UK", 90, 17, new int[] { 2008 }, new string[] { "McLaren" })); racers.Add(new Racer("Jenson", "Button", "UK", 208, 12, new int[] { 2009 }, new string[] { "Brawn GP" })); racers.Add(new Racer("Sebastian", "Vettel", "Germany", 81, 21, new int[] { 2010, 2011 }, new string[] { "Red Bull Racing" })); } return racers; } public struct Employee { /// <summary> /// 名字 /// </summary> public string Name; /// <summary> /// 年纪 /// </summary> public int Age; /// <summary> /// 性别 /// </summary> public string Gender; /// <summary> /// 薪水 /// </summary> public int Salary; } public struct Employee2 { /// <summary> /// 名字 /// </summary> public string Name; /// <summary> /// 语言 /// </summary> public string language; /// <summary> /// 城市 /// </summary> public string city; /// <summary> /// 已婚 /// </summary> public bool married; } public static List<Employee> employees = new List<Employee>() { new Employee(){ Age=40, Name="Peter Claus", Gender="Male", Salary=61000}, new Employee(){ Age=35, Name="Jose Mond", Gender="Male", Salary=62000}, new Employee(){ Age=38, Name="Helen Gant", Gender="Female", Salary=38000}, new Employee(){ Age=42, Name="Jo Parker", Gender="Male", Salary=52000}, new Employee(){ Age=22, Name="Alex Mueller", Gender="Male", Salary=39000}, new Employee(){ Age=53, Name="Abbi Black", Gender="female", Salary=56000}, new Employee(){ Age=51, Name="Mike Mockson", Gender="Male", Salary=82000} }; public static List<Employee2> employees2 = new List<Employee2>() { new Employee2(){ Name="Peter Claus", city="wu han", language="Chinese", married=false}, new Employee2(){ Name="Jose Mond", city="wu han", language="English", married=false}, new Employee2(){ Name="Helen Gant", city="chong qing", language="Chinese", married=false}, new Employee2(){ Name="Jo Parker", city="bei jing", language="Chinese", married=true}, new Employee2(){ Name="Alex Mueller", city="he fei", language="Chinese", married=false}, new Employee2(){ Name="Abbi Black", city="chong qing", language="Chinese", married=true}, new Employee2(){ Name="Mike Mockson", city="dong guang", language="English", married=true}, }; static Person p1 = new Person() { Name = "ABC", Age = 18 }; static Person p2 = new Person() { Name = "EFG", Age = 19 }; static Person p3 = new Person() { Name = "LMN", Age = 20 }; static Person p4 = new Person() { Name = "XYZ", Age = 21 }; static List<Person> pList = new List<Person> { p1, p2, p3, p4 }; static Department d1 = new Department() { Name = "A1", Employee = p1 }; static Department d2 = new Department() { Name = "A2", Employee = p2 }; static Department d3 = new Department() { Name = "A3", Employee = p1 }; static Department d4 = new Department() { Name = "B1", Employee = p3 }; static Department d5 = new Department() { Name = "B2", Employee = p4 }; static Department d6 = new Department() { Name = "B3", Employee = p4 }; static List<Department> dList = new List<Department> { d1, d2, d3, d4, d5, d6 }; public static ArrayList arylist = new ArrayList() { "Mango", "Orange", "Apple",32, new Employee(){ Age=46, Name="Peter blaus", Gender="Male", Salary=66000}, "Banana" }; static void printf<T>(IEnumerable<T> data, string msg="") { var sb1 = new StringBuilder(); if (data is IEnumerable<Employee>) { ((IEnumerable<Employee>)data).ToList().ForEach(s => sb1.Append($"{s.Age},{s.Name},{s.Gender},{s.Salary}\r\n")); } else if(data is IEnumerable<int> || data is IEnumerable<string>) { data.ToList().ForEach(s => sb1.Append(s.ToString() + "\r\n")); } else { foreach(var m in data) { sb1.Append(m.ToString()+"\r\n"); } } if(msg.Length>0) { Console.WriteLine($"{msg}----------------------------------"); } Console.WriteLine(sb1.ToString()); } static void Main(string[] args) { //过滤 printf(employees.Where((s) => s.Salary >= 52000), "过滤"); printf(arylist.OfType<int>()); //投影 printf(employees .Where(s => s.Name.StartsWith("J")) .Select(s => s), "投影"); //返回单列 printf(employees .Where(s => s.Name.StartsWith("J")) .Select(s => s.Name) .ToList()); //多列的匿名类 printf(employees .Where(s => s.Name.StartsWith("J")) .Select(s =>new { s.Name,s.Age,s.Salary }) ); //分区 //Skip:跳过序列中的指定位置之前的元素。 printf(employees.Skip(4), "分区"); //SkipWhile:基于谓词函数跳过元素,直到某元素不再满足条件。 printf(employees.SkipWhile(s => s.Age < 50)); //Take:提取序列中的指定位置之前的元素。 printf(employees.Take(2)); //TakeWhile:基于谓词函数提取元素,直到某元素不再满足条件。 printf(employees.TakeWhile(s => s.Age <50)); //连接 //Join:根据键选择器函数联接两个序列并提取值对。 printf(employees.Join(employees2, s => s.Name, s2 => s2.Name, (s, s2) => new { Name = s.Name, Age = s.Age, Gender = s.Gender, Salary = s.Salary, City = s2.city, Language = s2.language, Married = s2.married }), "分区"); //GroupJoin: 根据键选择器函数联接两个序列,并对每个元素的结果匹配项进行分组。 var result2 = pList.Join(dList, person => person, department => department.Employee, (person, department) => new { Person = person, Department = department }); foreach (var item1 in result2) { Console.Write($"Name:{item1.Person} & Department:{item1.Department} "); Console.WriteLine(); } Console.WriteLine(); var result= pList.GroupJoin(dList, person => person, department => department.Employee, (person, departments) => new { Person = person, Department = departments.Select(d => d) }); foreach (var item1 in result) { Console.Write($"Name:{item1.Person} & "); foreach (var item22 in item1.Department) { if (item1.Department.First() == item22) Console.Write($"Department:{item22} "); else Console.Write($"{item22} "); } Console.WriteLine(); } //串联 //排序 //分组 //集合 //转换 //等同 //元素 //生成 //数量 //聚集 //1、where子句 var racers = from r in GetChampions() where r.Wins > 15 && (r.Country == "Brazil" || r.Country == "Austria") select r; var item = GetChampions().Where(p => p.Wins > 15 && (p.Country == "Brazil" || p.Country == "Austria")).Select(r => r); //2、根据索引筛选 var racers1 = GetChampions(). Where((r, index) => r.LastName.StartsWith("A") && index % 2 != 0); //3、类型筛选 object[] data = { "one", 2, 3, "four", "five", 6 }; var query = data.OfType<string>(); //4、复合的from子句 var ferrariDrivers = from r in GetChampions() from c in r.Cars where c == "Ferrari" orderby r.LastName select r.FirstName + " " + r.LastName; var item2 = GetChampions().SelectMany(r => r.Cars, (r, c) => new { Racer = r, Car = c }). Where(r => r.Car == "Ferrari").OrderBy(r => r.Racer.LastName).Select(r => r.Racer.FirstName + "" + r.Racer.LastName); //5、排序 var racers3 = from r in GetChampions() where r.Country == "Brazil" orderby r.Wins descending select r; var racers2 = from r in GetChampions() orderby r.Country, r.LastName, r.FirstName select r; var racers4 = GetChampions().OrderBy(r => r.Country).ThenBy(r => r.LastName).ThenBy(r => r.FirstName); //6、分组 var countries = from r in GetChampions() group r by r.Country into g orderby g.Count() descending, g.Key where g.Count() >= 2 select new { Country = g.Key, Count = g.Count() }; var item5 = GetChampions().GroupBy(r => r.Country).OrderByDescending(p => p.Count()). ThenBy(p => p.Key).Where(p => p.Count() >= 2).Select(p => new { Country = p.Key, Count = p.Count() }); //7、内链接 var racers7 = from r in GetChampions() from y in r.Years select new { Year = y, Name = r.FirstName + " " + r.LastName }; //var teams7 = from t in GetContructorChampions() // from y in t.Years // select new // { // Year = y, // Name = t.Name // }; //8、左连接 //var racersAndTeams = // (from r in racers // join t in teams on r.Year equals t.Year into rt // from t in rt.DefaultIfEmpty() // orderby r.Year // select new // { // Year = r.Year, // Champion = r.Name, // Constructor = t == null ? "no constructor championship" : t.Name // }).Take(10); //9、zip 合并 var racerNames = from r in GetChampions() where r.Country == "Italy" orderby r.Wins descending select new { Name = r.FirstName + " " + r.LastName }; var racerNamesAndStarts = from r in GetChampions() where r.Country == "Italy" orderby r.Wins descending select new { LastName = r.LastName, Starts = r.Starts }; var racers9 = racerNames.Zip(racerNamesAndStarts, (first, second) => first.Name + ", starts: " + second.Starts); //10、聚合函数 var query10 = from r in GetChampions() let numberYears = r.Years.Count() where numberYears >= 3 orderby numberYears descending, r.LastName select new { Name = r.FirstName + " " + r.LastName, TimesChampion = numberYears }; var countries10 = (from c in from r in GetChampions() group r by r.Country into c select new { Country = c.Key, Wins = (from r1 in c select r1.Wins).Sum() } orderby c.Wins descending, c.Country select c).Take(5); //https://www.cnblogs.com/farmer-y/p/5977483.html Console.ReadLine(); } } public class Racer : IComparable<Racer>, IFormattable { public Racer() { } public Racer(string firstName, string lastName, string country, int starts, int wins) : this(firstName, lastName, country, starts, wins, null, null) { } public Racer(string firstName, string lastName, string country, int starts, int wins, IEnumerable<int> years, IEnumerable<string> cars) { this.FirstName = firstName; this.LastName = lastName; this.Country = country; this.Starts = starts; this.Wins = wins; this.Years = new List<int>(years); this.Cars = new List<string>(cars); } public string FirstName { get; set; } public string LastName { get; set; } public string Country { get; set; } public int Wins { get; set; } public int Starts { get; set; } public IEnumerable<string> Cars { get; private set; } public IEnumerable<int> Years { get; private set; } public override string ToString() { return String.Format("{0} {1}", FirstName, LastName); } public int CompareTo(Racer other) { if (other == null) return -1; return string.Compare(this.LastName, other.LastName); } public string ToString(string format) { return ToString(format, null); } public string ToString(string format, IFormatProvider formatProvider) { switch (format) { case null: case "N": return ToString(); case "F": return FirstName; case "L": return LastName; case "C": return Country; case "S": return Starts.ToString(); case "W": return Wins.ToString(); case "A": return String.Format("{0} {1}, {2}; starts: {3}, wins: {4}", FirstName, LastName, Country, Starts, Wins); default: throw new FormatException(String.Format("Format {0} not supported", format)); } } } }
参考资料:
C#中使用Join与GroupJoin将两个集合进行关联与分组 https://www.cnblogs.com/newcapecjmc/p/11193852.html
LINQ 标准查询操作概述 https://www.cnblogs.com/liqingwen/p/5801249.html
---------------------
作者:hackpig
来源:www.skcircle.com
版权声明:本文为博主原创文章,转载请附上博文链接!
本文出自勇哥的网站《少有人走的路》wwww.skcircle.com,转载请注明出处!讨论可扫码加群: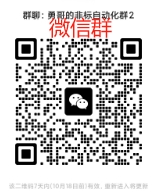
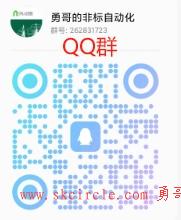
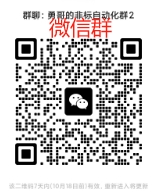
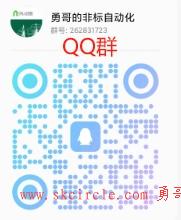