命名空间:netMarketing.http.httpClass
功能:httpClass类提供http的post,get方法,以及相关cookie的处理
功能详细说明:
这个类封装了http访问的方法,可以用于读取网页,提交网页,下载网络图片与文件,还可以带cookie进行网站登陆、发贴、上传图片与文件等操作。
这个类被设计成配合抓包工具,可以更方便的处理http的响应头。
常用函数列表:
public Image DowloadCheckImg(string url = "", string postOrGet = "GET", string proxy = "", StringBuilder protocolHeader = null, string postData = "") public bool Download(string url, string localfile) // Http方式下载文件 public bool HttpDownloadFile(string url, string filePath) // Http下载文件 public string sendWebRequet(string url = "", string postOrGet = "GET", string proxy = "", StringBuilder protocolHeader = null, string postData = "") // 发送web请求,可以是Get或者Post请求 public Encoding getEncoding() // 返回sendWebRequet使用的编码 public void setEncoding(Encoding encod) // 设置sendWebRequet使用的编码 public void setCookie(string url, string cookieStr) // 设置sendWebRequet时要用到的cookie public void setCookie(CookieContainer cookie) // 设置sendWebRequet时要用到的cookie public CookieContainer getCookie() // sendWebRequet后读取返回的cookie public string getCookie(string url, CookieContainer cookieObj = null) // 取字符串表示的Cookie键值 public static string getMidString(string html, string leftString, string rightString) // 取左右串之间的文本,取不到则返回空串 public string getCsrfToken(string html, string leftString = "Token=", string rightString = "\"") // 取CsrfToken字符串,通常网站处理跨站域请求伪造(CSRF),会在post提交时要求带上这个Token,它通常在登录时产生。 public string postImgOrFile(string url, StringBuilder protocolHeader, byte[] postdata) //POST上传图片或者文件 public byte[] readFileToByteAry(string filename) //读文件,转为字节数组byte[] public Image downImg(string imgurl) //下载图片,返回Image对象 public void downImg(string imgUrl, string saveAsPath) //下载网络图片 public static WebResponse GetResponseNoCache(Uri uri) //取网页Response,无缓存 public void strReplaceResByteAry(ref byte[] targetAry, string searchStr, byte[] replaceAry, Encoding encod) //文本替换,返回字节数组 public void replaceByteAry(ref Byte[] all, Byte[] s, Byte[] t) //字节数组替换 public void strReplaceResByteAry(ref byte[] targetAry, string searchStr, string replaceStr, Encoding encod) //文本替换,返回字节数组 public byte[] strReplaceResByteAry(string targetStr, string searchStr, string replaceStr, Encoding encod) //文本替换,返回字节数组 public string getRandomSpaceLine() //随机分隔符 public byte[] readByteAryFromDiskFile(string fileNamePath) //读byte[]从磁盘文件 public bool saveByteAryToDiskFile(string fileNamePath, byte[] byteAry) //写byte[]到磁盘文件 public string byteAryToString(byte[] data, Encoding encod) //由byte[]取字符串 public static int getPort(string url) public static string getHttpHost(string url) public static string getHost(string url)
参考例子:
下面的例子中,有关登陆的一些用户名与网址进行了隐私处理,你需要把它修改成自己测试用的网址。
其它的一些操作,例如自动发贴,上传图片等,由于隐私的原因就暂时不写在演示代码中了。
如果大家有需求的话,勇哥可以考虑写个相关的专题。
using netMarketing.http; using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Net; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace httpClassTest { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void button2_Click(object sender, EventArgs e) { //读网页 httpClass http = new httpClass(); var ary1=http.sendWebRequet("http://www.skcircle.com/"); richTextBox1.Text = ary1; } private void button3_Click(object sender, EventArgs e) { //读网络图片 httpClass http = new httpClass(); pictureBox1.Image= http.downImg("http://www.skcircle.com/zb_users/upload/2018/09/201809231537706956485696.png"); } private void button1_Click(object sender, EventArgs e) { //登陆 string token = ""; CookieContainer cookie; httpClass http = new httpClass(); //"btnPost=登录&username=用户名&password=436e29daba60789391c234c231f0fb17&savedate=1"; var url = "http://www.网站.com/zb_system/cmd.php?act=verify"; var postdata = string.Format("btnPost={0}&username={1}&password=436e29daba60789391c234c231f0fb17&savedate=1", "登录".UrlEncode(), "用户名".UrlEncode() ); //下面是抓包得到的需要提交的post数据 //postdata = "btnPost=%E7%99%BB%E5%BD%95&username=%E5%B1%66%E9%83%B3%E5%81%9C%E6%AD%A2&password=436e29daba60789391c234c231f0fb17&savedate=1"; var headinfo = new StringBuilder(); headinfo.AppendLine("Referer:http://www.网站.com/zb_system/login.php"); headinfo.AppendLine("Accept:text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8"); headinfo.AppendLine("Accept-Language:zh-CN,zh;q=0"); headinfo.AppendLine("User-Agent:Mozilla/5.0 (Windows NT 5.1) AppleWebKit/535.1 (KHTML, like Gecko) Chrome/14.0.835.202 Safari/535.1"); headinfo.AppendLine("Content-Type:application/x-www-form-urlencoded"); headinfo.AppendLine("Cookie:timezone=8"); richTextBox1.Text = http.sendWebRequet(url, "POST", "", headinfo, postdata); cookie = http.getCookie(); var s1 = http.getCookie(url); token = http.getCsrfToken(http.ResponseHtml); } } }
---------------------
作者:hackpig
来源:www.skcircle.com
版权声明:本文为博主原创文章,转载请附上博文链接!
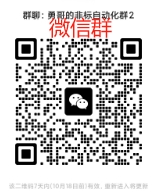
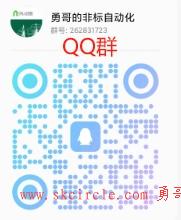