#1楼
#2楼
//Example from non UI thread - private void SaveAssetAsDraft() { SaveAssetDataAsDraft(); } private async Task<bool> SaveAssetDataAsDraft() { var id = await _assetServiceManager.SavePendingAssetAsDraft(); return true; } //UI Thread - var result = Task.Run(() => SaveAssetDataAsDraft().Result).Result;
#3楼
internal static class AsyncHelper { private static readonly TaskFactory _myTaskFactory = new TaskFactory(CancellationToken.None, TaskCreationOptions.None, TaskContinuationOptions.None, TaskScheduler.Default); public static TResult RunSync<TResult>(Func<Task<TResult>> func) { return AsyncHelper._myTaskFactory .StartNew<Task<TResult>>(func) .Unwrap<TResult>() .GetAwaiter() .GetResult(); } public static void RunSync(Func<Task> func) { AsyncHelper._myTaskFactory .StartNew<Task>(func) .Unwrap() .GetAwaiter() .GetResult(); } }
public static TUser FindById<TUser, TKey>(this UserManager<TUser, TKey> manager, TKey userId) where TUser : class, IUser<TKey> where TKey : IEquatable<TKey> { if (manager == null) { throw new ArgumentNullException("manager"); } return AsyncHelper.RunSync<TUser>(() => manager.FindByIdAsync(userId)); } public static bool IsInRole<TUser, TKey>(this UserManager<TUser, TKey> manager, TKey userId, string role) where TUser : class, IUser<TKey> where TKey : IEquatable<TKey> { if (manager == null) { throw new ArgumentNullException("manager"); } return AsyncHelper.RunSync<bool>(() => manager.IsInRoleAsync(userId, role)); }
#4楼
static void Main(string[] args) { MainAsync().GetAwaiter().GetResult(); } static async Task MainAsync() { /*await stuff here*/ }
#5楼
private void DeleteSynchronous(string path) { StorageFolder localFolder = Windows.Storage.ApplicationData.Current.LocalFolder; Task t = localFolder.DeleteAsync(StorageDeleteOption.PermanentDelete).AsTask(); t.Wait(); } private void FunctionThatNeedsToBeSynchronous() { // Do some work here // .... // Delete something in storage synchronously DeleteSynchronous("pathGoesHere"); // Do other work here // ..... }
#6楼
using System; using System.Collections.Concurrent; using System.Collections.Generic; using System.Threading; using System.Threading.Tasks; namespace Microsoft.Threading { /// <summary>Provides a pump that supports running asynchronous methods on the current thread.</summary> public static class AsyncPump { /// <summary>Runs the specified asynchronous method.</summary> /// <param name="asyncMethod">The asynchronous method to execute.</param> public static void Run(Action asyncMethod) { if (asyncMethod == null) throw new ArgumentNullException("asyncMethod"); var prevCtx = SynchronizationContext.Current; try { // Establish the new context var syncCtx = new SingleThreadSynchronizationContext(true); SynchronizationContext.SetSynchronizationContext(syncCtx); // Invoke the function syncCtx.OperationStarted(); asyncMethod(); syncCtx.OperationCompleted(); // Pump continuations and propagate any exceptions syncCtx.RunOnCurrentThread(); } finally { SynchronizationContext.SetSynchronizationContext(prevCtx); } } /// <summary>Runs the specified asynchronous method.</summary> /// <param name="asyncMethod">The asynchronous method to execute.</param> public static void Run(Func<Task> asyncMethod) { if (asyncMethod == null) throw new ArgumentNullException("asyncMethod"); var prevCtx = SynchronizationContext.Current; try { // Establish the new context var syncCtx = new SingleThreadSynchronizationContext(false); SynchronizationContext.SetSynchronizationContext(syncCtx); // Invoke the function and alert the context to when it completes var t = asyncMethod(); if (t == null) throw new InvalidOperationException("No task provided."); t.ContinueWith(delegate { syncCtx.Complete(); }, TaskScheduler.Default); // Pump continuations and propagate any exceptions syncCtx.RunOnCurrentThread(); t.GetAwaiter().GetResult(); } finally { SynchronizationContext.SetSynchronizationContext(prevCtx); } } /// <summary>Runs the specified asynchronous method.</summary> /// <param name="asyncMethod">The asynchronous method to execute.</param> public static T Run<T>(Func<Task<T>> asyncMethod) { if (asyncMethod == null) throw new ArgumentNullException("asyncMethod"); var prevCtx = SynchronizationContext.Current; try { // Establish the new context var syncCtx = new SingleThreadSynchronizationContext(false); SynchronizationContext.SetSynchronizationContext(syncCtx); // Invoke the function and alert the context to when it completes var t = asyncMethod(); if (t == null) throw new InvalidOperationException("No task provided."); t.ContinueWith(delegate { syncCtx.Complete(); }, TaskScheduler.Default); // Pump continuations and propagate any exceptions syncCtx.RunOnCurrentThread(); return t.GetAwaiter().GetResult(); } finally { SynchronizationContext.SetSynchronizationContext(prevCtx); } } /// <summary>Provides a SynchronizationContext that's single-threaded.</summary> private sealed class SingleThreadSynchronizationContext : SynchronizationContext { /// <summary>The queue of work items.</summary> private readonly BlockingCollection<KeyValuePair<SendOrPostCallback, object>> m_queue = new BlockingCollection<KeyValuePair<SendOrPostCallback, object>>(); /// <summary>The processing thread.</summary> private readonly Thread m_thread = Thread.CurrentThread; /// <summary>The number of outstanding operations.</summary> private int m_operationCount = 0; /// <summary>Whether to track operations m_operationCount.</summary> private readonly bool m_trackOperations; /// <summary>Initializes the context.</summary> /// <param name="trackOperations">Whether to track operation count.</param> internal SingleThreadSynchronizationContext(bool trackOperations) { m_trackOperations = trackOperations; } /// <summary>Dispatches an asynchronous message to the synchronization context.</summary> /// <param name="d">The System.Threading.SendOrPostCallback delegate to call.</param> /// <param name="state">The object passed to the delegate.</param> public override void Post(SendOrPostCallback d, object state) { if (d == null) throw new ArgumentNullException("d"); m_queue.Add(new KeyValuePair<SendOrPostCallback, object>(d, state)); } /// <summary>Not supported.</summary> public override void Send(SendOrPostCallback d, object state) { throw new NotSupportedException("Synchronously sending is not supported."); } /// <summary>Runs an loop to process all queued work items.</summary> public void RunOnCurrentThread() { foreach (var workItem in m_queue.GetConsumingEnumerable()) workItem.Key(workItem.Value); } /// <summary>Notifies the context that no more work will arrive.</summary> public void Complete() { m_queue.CompleteAdding(); } /// <summary>Invoked when an async operation is started.</summary> public override void OperationStarted() { if (m_trackOperations) Interlocked.Increment(ref m_operationCount); } /// <summary>Invoked when an async operation is completed.</summary> public override void OperationCompleted() { if (m_trackOperations && Interlocked.Decrement(ref m_operationCount) == 0) Complete(); } } } }
AsyncPump.Run(() => FooAsync(...));
#7楼
public class LogReader { ILogger _logger; public LogReader(ILogger logger) { _logger = logger; } public LogEntity GetLog() { Task<LogEntity> task = Task.Run<LogEntity>(async () => await GetLogAsync()); return task.Result; } public async Task<LogEntity> GetLogAsync() { var result = await _logger.GetAsync(); // more code here... return result as LogEntity; } }
#8楼
var result = Task.Run(async () => await configManager.GetConfigurationAsync()).ConfigureAwait(false); OpenIdConnectConfiguration config = result.GetAwaiter().GetResult();
var result=result.GetAwaiter().GetResult().AccessToken
#9楼
#10楼
public static class AsyncHelpers { private static readonly TaskFactory taskFactory = new TaskFactory(CancellationToken.None, TaskCreationOptions.None, TaskContinuationOptions.None, TaskScheduler.Default); /// <summary> /// Executes an async Task method which has a void return value synchronously /// USAGE: AsyncUtil.RunSync(() => AsyncMethod()); /// </summary> /// <param name="task">Task method to execute</param> public static void RunSync(Func<Task> task) => taskFactory .StartNew(task) .Unwrap() .GetAwaiter() .GetResult(); /// <summary> /// Executes an async Task<T> method which has a T return type synchronously /// USAGE: T result = AsyncUtil.RunSync(() => AsyncMethod<T>()); /// </summary> /// <typeparam name="TResult">Return Type</typeparam> /// <param name="task">Task<T> method to execute</param> /// <returns></returns> public static TResult RunSync<TResult>(Func<Task<TResult>> task) => taskFactory .StartNew(task) .Unwrap() .GetAwaiter() .GetResult(); }
var t = AsyncUtil.RunSync<T>(() => AsyncMethod<T>());
#11楼
MethodAsync().RunSynchronously()
#12楼
#13楼
public async Task<string> StartMyTask() { await Foo() // code to execute once foo is done } static void Main() { var myTask = StartMyTask(); // call your method which will return control once it hits await // now you can continue executing code here string result = myTask.Result; // wait for the task to complete to continue // use result }
#14楼
var task = MyAsyncMethod(); var result = task.WaitAndUnwrapException();
var result = AsyncContext.RunTask(MyAsyncMethod).Result;
var result = AsyncContext.Run(MyAsyncMethod);
同步方法调用异步方法,获得
Task
。同步方法对
Task
进行阻塞等待。async
方法使用await
而不使用ConfigureAwait
。在这种情况下,
Task
无法完成,因为它仅在async
方法完成时才完成;async
方法无法完成,因为它正在尝试安排其继续到SynchronizationContext
,并且WinForms / WPF / SL / ASP.NET将不允许继续运行,因为同步方法已在该上下文中运行。
var task = Task.Run(async () => await MyAsyncMethod()); var result = task.WaitAndUnwrapException();
本文出自勇哥的网站《少有人走的路》wwww.skcircle.com,转载请注明出处!讨论可扫码加群: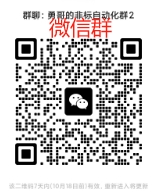
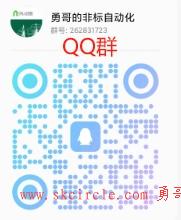
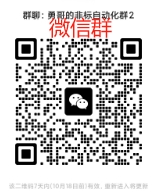
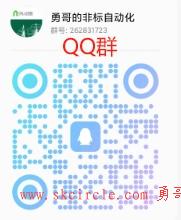