命名空间:netMarketing.automation.Robot.Epson
功能:Epson机器人的远程控制类
功能详细说明:
EpsonRobot类是一个用于以太网远程控制Epson机器人的类。
使用前,先把机器人的控制器配置为“远程以太网”模式。
有关远程控制的知识,请参考勇哥另一篇贴子的介绍:
Epson机器人远程控制指令测试
注意:使用这个类需要使用netMarketing V1.3.0及以上的版本。
常用函数列表:
/// 配置 public ConfigBase Config { get { return config; } } /// IO输入配置 public IO24Config InConfig { get { return inConfig; } set { inConfig = value; } } /// IO输出配置 public IO16Config OutConfig { get { return outConfig; } set { outConfig = value; } } /// 设备状态 public Status Status { get { return status; } } /// 连接状态 public bool IsConnected { get { return isConnected; } } /// 登陆状态 public bool IsLogin { get { return isLogin; } set { isLogin = value; } } /// 是否在执行命令 public bool IsExeCMD { get { return isExeCMD; } set { isExeCMD = value; } } /// 设置工具坐标 public int ToolNo public EpsonRobot(int id, string name) : base(id, name) /// 初始化 public bool Init() /// 暂停 public bool? Pause() /// 连接 public bool Connect() /// 关闭连接 public void Close() /// 登陆 public bool Login() /// 登出 public bool LoginOut() /// 执行命令 public bool? ExecuteCMD(ERemotCMD cmd, params string[] param) /// <summary> /// 爱普生机器人远程控制命令 /// </summary> public enum ERemotCMD { /// <summary> ///登陆 /// </summary> Login, /// <summary> /// 登出 /// </summary> Logout, /// <summary> /// 执行指定编号的函数 /// </summary> Start, /// <summary> /// 停止所有的任务和命令 /// </summary> Stop, /// <summary> /// 暂停所有任务 /// </summary> Pause, /// <summary> /// 继续暂停了的任务 /// </summary> Continue, /// <summary> /// 清除紧急停止和错误 /// </summary> Reset, /// <summary> /// 打开机器人电机 /// </summary> SetMotorsOn, /// <summary> /// 关闭机器人电机 /// </summary> SetMotorsOff, /// <summary> /// 选择机器人 /// </summary> SetCurRobot, /// <summary> /// 过去当前的机器人编号 /// </summary> GetCurRobot, /// <summary> /// 将机器人手臂移动到由用户定义的起始点位置上 /// </summary> Home, /// <summary> /// 获取指定的I/O位 /// </summary> GetIO, /// <summary> /// 设置I/O指定位 /// </summary> SetIO, /// <summary> /// 获得指定的I/O端口(8位 /// </summary> GetIOByte, /// <summary> /// 设置I/O指定端口(8位) /// </summary> SetIOByte, /// <summary> /// 获得指定的I/O字端口(16位) /// </summary> GetIOWord, /// <summary> /// 设置I/O指定字端口(8位) /// </summary> SetIOWord, /// <summary> /// 获取指定的内存I/O位 /// </summary> GetMemIO, /// <summary> /// 设置指定的内存I/O位 /// </summary> SetMemIO, /// <summary> /// 获取指定内存I/O端口 /// </summary> GetMemIOByte, /// <summary> /// 设置指定的内存I/O端口(8位) /// </summary> SetMemIOByte, /// <summary> /// 获取指定的内存I/O字端口(16位) /// </summary> GetMemIOWord, /// <summary> /// 设置指定的内存I/O字端口(16位) /// </summary> SetMemIOWord, /// <summary> /// 获取备份(全局保留)参数的值 /// </summary> GetVariable, /// <summary> /// 获取备份(全局保留)数组参数的值 /// </summary> SetVariable, /// <summary> /// 获取控制器的状态 /// </summary> GetStatus, /// <summary> /// 执行命令 /// </summary> Execute, /// <summary> /// 中止命令的执行 /// </summary> Abort, /// <summary> /// 空命令 /// </summary> NULL, }
参考例子:
using netMarketing.automation.baseClass; using netMarketing.automation.hardware.Light; using netMarketing.automation.Robot.Epson; using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace demo { public partial class Form1 : Form { EpsonRobot RobotBusines = new EpsonRobot(123, "epson"); public Form1() { InitializeComponent(); NotifyG.EventHandlerNotify += NotifyG_EventHandlerNotify; RobotBusines.Config.Folder = "Robot"; RobotBusines.Config.Name = "epson"; } private void NotifyG_EventHandlerNotify(object sender, NotifyEventArgs e) { rtbMsg.AppendText(e.Message); } private void Form1_Load(object sender, EventArgs e) { } private void button1_Click(object sender, EventArgs e) { rtbMsg.AppendText($"登陆:{RobotBusines.Login()}\r\n"); } private void btnSave_Click(object sender, EventArgs e) { rtbMsg.AppendText($"参数保存:{ RobotBusines.Config.Save()}\r\n"); } private void button2_Click(object sender, EventArgs e) { rtbMsg.AppendText($"登出:{RobotBusines.LoginOut()}\r\n"); } private void button3_Click(object sender, EventArgs e) { RobotBusines.ExecuteCMD(ERemotCMD.SetMotorsOn, "1"); } private void button8_Click(object sender, EventArgs e) { rtbMsg.AppendText($"类初始化:{RobotBusines.Init()}\r\n"); propertyGrid1.SelectedObject = RobotBusines.Config; } private void button9_Click(object sender, EventArgs e) { RobotBusines.Pause(); } private void button10_Click(object sender, EventArgs e) { RobotBusines.ExecuteCMD(ERemotCMD.Continue,"1"); } private void button4_Click(object sender, EventArgs e) { RobotBusines.ExecuteCMD(ERemotCMD.SetMotorsOff, "1"); } private void button5_Click(object sender, EventArgs e) { RobotBusines.ExecuteCMD(ERemotCMD.Execute, "Go XY(0, 450, 260, 90, 0, 180)"); } private void button6_Click(object sender, EventArgs e) { RobotBusines.ExecuteCMD(ERemotCMD.Execute, "Go XY(0, 250, 360, 110, 0, 120)"); } private void button7_Click(object sender, EventArgs e) { RobotBusines.ExecuteCMD(ERemotCMD.Execute, "print RealPos"); } } }
---------------------
作者:hackpig
来源:www.skcircle.com
版权声明:本文为博主原创文章,转载请附上博文链接!
本文出自勇哥的网站《少有人走的路》wwww.skcircle.com,转载请注明出处!讨论可扫码加群: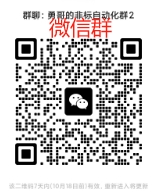
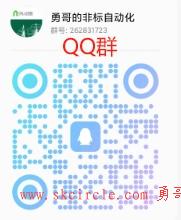
本帖最后由 勇哥,很想停止 于 2019-07-18 15:34:49 编辑 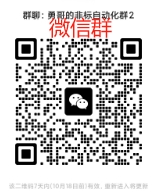
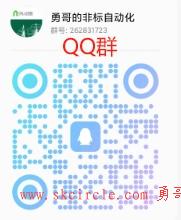