MVC模式主要解决的问题就是将表示层和业务层进行分离,在以往做WINFORM项目的时候,通常都是将很多的逻辑代码直接写在了Form.cs代码的事件里,这样的话业务逻辑就和界面紧耦合在一起了,现在我们采用MVC来解耦。
首先建立Model:
[csharp] view plain copy using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.ComponentModel; namespace WindowsFormsApplication10 { public class Person : INotifyPropertyChanged { private string _id; public string ID { get { return _id; } set { _id = value; OnPropertyChanged("ID"); } } private string _name; public string Name { get { return _name; } set { _name = value; OnPropertyChanged("Name"); } } #region INotifyPropertyChanged 成员 public event PropertyChangedEventHandler PropertyChanged; protected void OnPropertyChanged(string PropertyName) { PropertyChangedEventHandler handler = PropertyChanged; if (handler != null) { handler(this, new PropertyChangedEventArgs(PropertyName)); } } #endregion } }
[csharp] view plain copy using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace WindowsFormsApplication10 { public class PersonControllor { public PersonForm View; public Person Model; public PersonControllor(PersonForm view) { //初始化了一个Model Model = new Person() { ID = "1", Name = "xiaojun" }; //通过构造函数将View注入到Controllor中 this.View = view; //建立起View 和Controllor的关联 //这时候View中能使用它所对应的Controllor进行业务逻辑的操作,Model也能和VIEW UI控件进行双向绑定 this.View.Controllor = this; } /// <summary> /// 执行一个业务逻辑 /// </summary> public void UpdatePerson() { UpdateToDataBase(Model); } private void UpdateToDataBase(Person p) { //do some thing //执行将数据插入到数据库的操作 System.Windows.Forms.MessageBox.Show("ID:" + p.ID + " Name:" + p.Name); } //这边的业务逻辑应该写在model里比较合适 } }
然后是View的实现:
[csharp] view plain copy using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace WindowsFormsApplication10 { public partial class PersonForm : Form { private PersonControllor _controllor; public PersonControllor Controllor { get { return _controllor; } set { this._controllor = value; //绑定一定只能写在给Controllor赋值以后而不能写在PersonForm的构造函数中(此时Controllor还未被实例化) //因为我们这里采用的是Controllor-First而不是View-First,不然Controllor.Model为null会异常 //将View通过构造函数注入到Controllor中的属于Controllor-First,这时候Controllor先创建 //将Controllor通过构造函数注入到View中的属于View-First,这时候View先创建 this.textBox1.DataBindings.Add("Text", Controllor.Model, "ID"); this.textBox2.DataBindings.Add("Text", Controllor.Model, "Name"); } } public PersonForm() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { //改变VIEW的UI控件的值,Controllor的Model会跟着变 this.textBox1.Text = "2"; this.textBox2.Text = "jacky"; Controllor.UpdatePerson(); } private void button2_Click(object sender, EventArgs e) { //改变Controllor的Model的值,VIEW的UI控件的值会跟着变 Controllor.Model.ID = "2"; Controllor.Model.Name = "jacky"; Controllor.UpdatePerson(); } private void PersonForm_Load(object sender, EventArgs e) { } } }
最后是程序启动:
[csharp] view plain copy using System; using System.Collections.Generic; using System.Linq; using System.Windows.Forms; namespace WindowsFormsApplication10 { static class Program { /// <summary> /// 应用程序的主入口点。 /// </summary> [STAThread] static void Main() { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); //Controllor-First模式,先创建Controllor(PersonControllor)再将View(PersonForm)注入到Controllor(PersonControllor)中 PersonControllor controllor = new PersonControllor(new PersonForm()); Application.Run(controllor.View); } } }
本文出自勇哥的网站《少有人走的路》wwww.skcircle.com,转载请注明出处!讨论可扫码加群: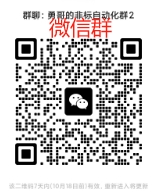
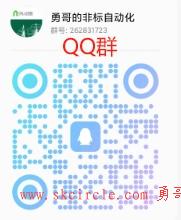
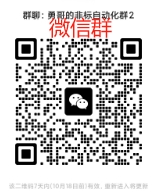
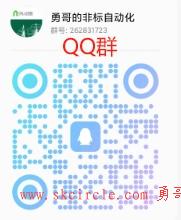