void AddEvent() { hWindowControl2.HMouseWheel += HWindowControl1_HMouseWheel; hWindowControl2.HMouseMove += HWindowControl1_HMouseMove; hWindowControl2.HMouseDown += HWindowControl1_HMouseDown; hWindowControl2.HMouseUp += HWindowControl1_HMouseUp; hWindowControl2.MouseLeave += HWindowControl1_MouseLeave; }
bool mousePressed = false; Point startPoint = Point.Empty; private void HWindowControl1_HMouseDown(object sender, HMouseEventArgs e) { mousePressed = true; startPoint = new Point((int)e.X, (int)e.Y); this.hWindowControl1.Cursor = Cursors.SizeAll; }
MouseMove 平移:注意关键之处,每次移动后的步进位置,需要重新给startPoint赋值,此处调试一下代码就知道如何写了。
private void HWindowControl1_HMouseMove(object sender, HMouseEventArgs e) { if (hMatrix == null || !mousePressed) return; HTuple hv_Matrix = null; double x = e.X - startPoint.X; double y = e.Y - startPoint.Y; ImageHandle.MoveImage(h_Image, out h_ScaledImage, hWindow, hMatrix, out hv_Matrix, y, x); startPoint = new Point((int)e.X, (int)e.Y); hMatrix = hv_Matrix; }
private void HWindowControl1_MouseLeave(object sender, EventArgs e) { mousePressed = false; startPoint = Point.Empty; this.hWindowControl1.Cursor = Cursors.Default; }
private void HWindowControl1_HMouseUp(object sender, HMouseEventArgs e) { mousePressed = false; startPoint = Point.Empty; this.hWindowControl1.Cursor = Cursors.Default; }
double _scaleValue = 1; HTuple hMatrix; //HTuple hScaleMatrix; private void HWindowControl1_HMouseWheel(object sender, HMouseEventArgs e) { if (e.Delta == 0) return; //获取鼠标在缩放之前的目标上的位置 //Point targetZoomFocus1 = e.GetPosition(this.hWindowControl1); double d = e.Delta / Math.Abs(e.Delta); if (_scaleValue < 0.5 && d < 0) return; if (_scaleValue > 5 && d > 0) return; double targetScaleValue = 0; _scaleValue += d * 0.2; HTuple hv_Matrix = null; if (d > 0) { targetScaleValue = 1 + 0.2; } else { targetScaleValue = 1 - 0.2; } ImageHandle.ScaleImage(h_Image, out h_ScaledImage, hWindow, hMatrix, out hv_Matrix, targetScaleValue, e.X, e.Y); hMatrix = hv_Matrix; }
public static void DrawRegion(HObject ho_Image, HWindow hWindow, out HTuple row1, out HTuple col1, out HTuple row2, out HTuple col2) { HObject ho_ROI_0; HOperatorSet.GenEmptyObj(out ho_ROI_0); HOperatorSet.SetColor(hWindow, "green"); HOperatorSet.DrawRectangle1(hWindow, out row1, out col1, out row2, out col2); HOperatorSet.GenRectangle1(out ho_ROI_0, row1, col1, row2, col2); HOperatorSet.DispObj(ho_ROI_0, hWindow); ho_ROI_0.Dispose(); }
public static void SaveModel(HObject ho_Image, HWindow hWindow, HTuple hMatrix, HTuple row1, HTuple col1, HTuple row2, HTuple col2, HTuple modelPath) { // Local iconic variables HObject ho_ROI_0, ho_TemplateImage, ho_ROI_1; HTuple hv_ModelID = null; HTuple hv_HomMat2D = null; HOperatorSet.GenEmptyObj(out ho_ROI_0); HOperatorSet.GenEmptyObj(out ho_ROI_1); HOperatorSet.GenEmptyObj(out ho_TemplateImage); HTuple hv_targetMatrix = null; HTuple hv_HomMat2D_Region = null; ho_TemplateImage.Dispose(); ho_ROI_0.Dispose(); HOperatorSet.GenRectangle1(out ho_ROI_0, row1, col1, row2, col2); HTuple hv_Sx = null, hv_Sy = null; HTuple hv_Phi = null, hv_Theta = null, hv_Tx = null, hv_Ty = null; HOperatorSet.HomMat2dToAffinePar(hMatrix, out hv_Sx, out hv_Sy, out hv_Phi, out hv_Theta, out hv_Tx, out hv_Ty); HTuple hv_HomMat2DIdentity = null; HOperatorSet.HomMat2dIdentity(out hv_HomMat2DIdentity); HOperatorSet.VectorAngleToRigid(0, 0, 0, -hv_Tx, -hv_Ty, 0, out hv_HomMat2D); //把图片变回去 HOperatorSet.HomMat2dScale(hv_HomMat2DIdentity, 1 / hv_Sx, 1 / hv_Sy, 0, 0, out hv_HomMat2D_Region); HOperatorSet.HomMat2dCompose(hv_HomMat2D_Region, hv_HomMat2D, out hv_targetMatrix); HOperatorSet.AffineTransRegion(ho_ROI_0, out ho_ROI_1, hv_targetMatrix, "nearest_neighbor"); HOperatorSet.ReduceDomain(ho_Image, ho_ROI_1, out ho_TemplateImage); HOperatorSet.ClearWindow(hWindow); HOperatorSet.DispObj(ho_TemplateImage, hWindow); HOperatorSet.CreateShapeModel(ho_TemplateImage, 5, (new HTuple(0)).TupleRad() , (new HTuple(360)).TupleRad(), (new HTuple(0.1406)).TupleRad(), (new HTuple("point_reduction_high")).TupleConcat( "no_pregeneration"), "use_polarity", ((new HTuple(48)).TupleConcat(60)).TupleConcat( 9), 3, out hv_ModelID); HOperatorSet.WriteShapeModel(hv_ModelID, modelPath); ho_Image.Dispose(); //ho_ROI_0.Dispose(); //ho_TemplateImage.Dispose(); }
本文出自勇哥的网站《少有人走的路》wwww.skcircle.com,转载请注明出处!讨论可扫码加群: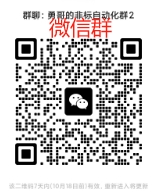
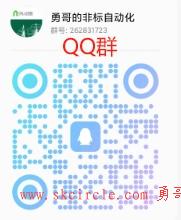
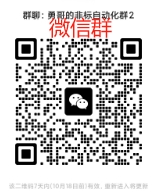
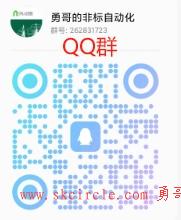