下面的代码示例演示如何实现 INotifyPropertyChanged 接口。 在 Windows 窗体数据绑定中使用的业务对象上实现此接口。 实现时,接口会与绑定控件通信,属性会对业务对象进行更改。
示例:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Drawing; using System.Runtime.CompilerServices; using System.Windows.Forms; // Change the namespace to the project name. namespace TestNotifyPropertyChangedCS { // This form demonstrates using a BindingSource to bind // a list to a DataGridView control. The list does not // raise change notifications. However the DemoCustomer type // in the list does. public partial class Form1 : Form { // This button causes the value of a list element to be changed. private Button changeItemBtn = new Button(); // This DataGridView control displays the contents of the list. private DataGridView customersDataGridView = new DataGridView(); // This BindingSource binds the list to the DataGridView control. private BindingSource customersBindingSource = new BindingSource(); public Form1() { InitializeComponent(); // Set up the "Change Item" button. this.changeItemBtn.Text = "Change Item"; this.changeItemBtn.Dock = DockStyle.Bottom; this.changeItemBtn.Click += new EventHandler(changeItemBtn_Click); this.Controls.Add(this.changeItemBtn); // Set up the DataGridView. customersDataGridView.Dock = DockStyle.Top; this.Controls.Add(customersDataGridView); this.Size = new Size(400, 200); } private void Form1_Load(object sender, EventArgs e) { // Create and populate the list of DemoCustomer objects // which will supply data to the DataGridView. BindingList<DemoCustomer> customerList = new BindingList<DemoCustomer>(); customerList.Add(DemoCustomer.CreateNewCustomer()); customerList.Add(DemoCustomer.CreateNewCustomer()); customerList.Add(DemoCustomer.CreateNewCustomer()); // Bind the list to the BindingSource. this.customersBindingSource.DataSource = customerList; // Attach the BindingSource to the DataGridView. this.customersDataGridView.DataSource = this.customersBindingSource; } // Change the value of the CompanyName property for the first // item in the list when the "Change Item" button is clicked. void changeItemBtn_Click(object sender, EventArgs e) { // Get a reference to the list from the BindingSource. BindingList<DemoCustomer> customerList = this.customersBindingSource.DataSource as BindingList<DemoCustomer>; // Change the value of the CompanyName property for the // first item in the list. customerList[0].CustomerName = "Tailspin Toys"; customerList[0].PhoneNumber = "(708)555-0150"; } } // This is a simple customer class that // implements the IPropertyChange interface. public class DemoCustomer : INotifyPropertyChanged { // These fields hold the values for the public properties. private Guid idValue = Guid.NewGuid(); private string customerNameValue = String.Empty; private string phoneNumberValue = String.Empty; public event PropertyChangedEventHandler PropertyChanged; // This method is called by the Set accessor of each property. // The CallerMemberName attribute that is applied to the optional propertyName // parameter causes the property name of the caller to be substituted as an argument. private void NotifyPropertyChanged([CallerMemberName] String propertyName = "") { if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs(propertyName)); } } // The constructor is private to enforce the factory pattern. private DemoCustomer() { customerNameValue = "Customer"; phoneNumberValue = "(312)555-0100"; } // This is the public factory method. public static DemoCustomer CreateNewCustomer() { return new DemoCustomer(); } // This property represents an ID, suitable // for use as a primary key in a database. public Guid ID { get { return this.idValue; } } public string CustomerName { get { return this.customerNameValue; } set { if (value != this.customerNameValue) { this.customerNameValue = value; NotifyPropertyChanged(); } } } public string PhoneNumber { get { return this.phoneNumberValue; } set { if (value != this.phoneNumberValue) { this.phoneNumberValue = value; NotifyPropertyChanged(); } } } } }
运行后,红框处的内容被替换。
此文章属于在线文档一部分,讲的主题是:windows窗体
有些主题很有趣。
各位有兴趣可以去看看。
本文出自勇哥的网站《少有人走的路》wwww.skcircle.com,转载请注明出处!讨论可扫码加群: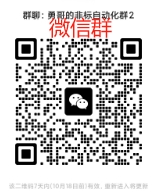
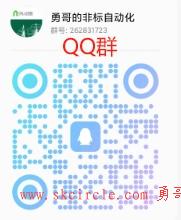
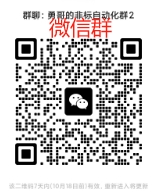
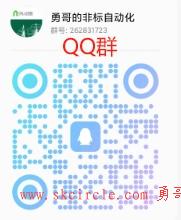