这是之前勇哥学习委托时的实验代码,有兴趣的朋友可以参考一下。
放在网页上比放在硬盘上睡觉要好些,翻阅起来也方便,对于这类常学常忘的内容,以后还是多整理一些放到网站上来。
演示程序的工程结构:
Class1.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace delegateTest { class myclass { private int value;//声明value私有字段 public int Value//声明只读属性 { get { return value; } } public myclass(int value)//构造函数 { this.value = value; } public static explicit operator int(myclass mc)//显示声明的myclass转int类处理方法 { return mc.value; } public static implicit operator myclass(int value)//隐式声明的int转myclass类处理方法 { return new myclass(value); } public static implicit operator string(myclass mc)//隐式声明的myclass转string类处理方法 { return ("定义的myclass类string类型转化结果"); } } }
Class2.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace delegateTest { public delegate double DoubleFun(double num); class doubleMethod { public double d1(double num) { return num * 8; } public double d2(double num) { return num * num; } } class Class2 { public ChangeUI onChange = null; DoubleFun[] df = { new doubleMethod().d1, new doubleMethod().d2 }; public void d3() { foreach (DoubleFun m in df) { d4(m, 1.32); d4(m, 7.49); d4(m, 1.414); } for (int i = 0; i < df.Length; i++) { d4(df[i], 1.32); d4(df[i], 7.49); d4(df[i], 1.414); } } public void d4(DoubleFun m, double value) { onChange(m.Invoke(value)); onChange(m(value)); } } }
Class3.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace delegateTest { class Employee { private string name; public string Name { get { return name; } set { name = value; } } private double salary; public double Salary { get { return salary; } set { salary = value; } } public Employee(string name, double salary) { this.name = name; this.salary = salary; } public static bool compare(Employee p1, Employee p2) { if (p1.Salary > p2.Salary) return true; return false; } } class Class3 { public SortFun<Employee> sortDelegate = null; //范型的冒泡排序 public void sort1<T>(IList<T> sortAry,Func<T,T,bool>comparison) { bool isok; do { isok = false; for (int i = 0; i < sortAry.Count - 1; i++) { if (comparison(sortAry[i], sortAry[i + 1])) { T tmp = sortAry[i]; sortAry[i] = sortAry[i + 1]; sortAry[i + 1] = tmp; isok = true; } } } while (isok); } //整形的冒泡排序 public void sort(ref int[] intary) { bool isok; do { isok = false; int tmp = 0; for (int i = 0; i < intary.Length - 1; i++) { if (intary[i] > intary[i + 1]) { tmp = intary[i]; intary[i] = intary[i + 1]; intary[i + 1] = tmp; isok = true; } } } while (isok); } } }
Class4.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace delegateTest { class fun7 { private double v1; public double V1 { get { return v1; } set { v1 = value; } } private double v2; public double V2 { get { return v2; } set { v2 = value; } } public void cal1(double num) { this.v1= num * 8; } public void cal2(double num) { this.v2= num * num; } } class fun8 { private double v1; public double V1 { get { return v1; } set { v1 = value; } } private double v2; public double V2 { get { return v2; } set { v2 = value; } } public void cal1(double num) { this.v1 = num * 8; throw new Exception("error!"); } public void cal2(double num) { this.v2 = num * num; } } class Class4 { public ChangeUI onChange = null; public void calfun() { fun7 f7 = new fun7(); Action<double> op = f7.cal1; op += f7.cal2; dis(op, 3.14,f7); } public void dis(Action<double> ac, double num,fun7 f7) { ac(num); onChange(f7.V1); onChange(f7.V2); } } class Class5 { public ChangeUI onChange = null; public void calfun() { fun8 f8 = new fun8(); Action<double> op = f8.cal1; op += f8.cal2; Delegate[] list1 = op.GetInvocationList(); foreach (Action<double> m in list1) { try { dis(m,3.14,f8); } catch { onChange(-999); } } } public void dis(Action<double> ac, double num, fun8 f7) { ac(num); if(ac.Method.ToString().IndexOf("cal1")>0) onChange(f7.V1); if(ac.Method.ToString().IndexOf("cal2")>0) onChange(f7.V2); } } }
Class5.cs
Class6.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace delegateTest { class CarInfoEventArgs:EventArgs { public string Car { get; private set; } public CarInfoEventArgs(string car) { this.Car = car; } } class Consumer { public ChangeUIstr onChange = null; private string name; public Consumer(string name) { this.name=name; } public void NewCarIsHere(object sender, CarInfoEventArgs e) { onChange(string.Format("{0}: car {1} is new",name,e.Car)); } } class CarDealer { public ChangeUIstr onChange = null; public event EventHandler<CarInfoEventArgs> NewCarInfo; public void NewCar(string car) { onChange(string.Format("CarDealer, new car {0}",car)); if (NewCarInfo != null) { NewCarInfo(this,new CarInfoEventArgs(car)); } } } }
Class7.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Windows; namespace rouEvent { class CarInfoEventArgs:EventArgs { private string _carname; public string Carname { get { return _carname; } set { _carname = value; } } public CarInfoEventArgs(string carname) { this._carname=carname; } } class Consumer { public delegateTest.ChangeUIstr onChange = null; private string _name; public string Name { get { return _name; } set { _name = value; } } public Consumer(string name) { this._name = name; } public void SubscriptionNewCarInfo(object ob, CarInfoEventArgs info) { onChange(string.Format("{0} 汽车商发布了新车: {1}",this._name,info.Carname)); } } class CarDealer { public event EventHandler<CarInfoEventArgs> NewCarEvent; public void PulishNewCarInfo(string NewCar) { NewCarEvent(this, new CarInfoEventArgs(NewCar)); } } class WeakCarInfoEventManager { } }
Class8.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace delegateTest { class Class8 { public delegate void ExceptionDelegate(string msg); public event ExceptionDelegate OnException; public void cal(int x, int y) { OnException(string.Format("{0},{1} error!",x,y)); } } }
Class9.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace delegateTest { public class delegateTest { public void funTest() { var list = new List<int>(); list.AddRange(new int[] { 2, 33, 21, 5, 71, 1, 88, 100, 32, 18, 19 }); var fun1 = new Func<int, bool>(target); IEnumerator<int> ie = list.Where<int>(fun1).GetEnumerator(); while (ie.MoveNext()) { Console.WriteLine(ie.Current.ToString()); } } public void funTest2() { Func<int, bool> fun; fun = s => s > 10; Console.WriteLine(fun(12)); fun = (s) => { return s > 10; }; Console.WriteLine(fun(9)); fun = delegate(int i) { return i > 10; }; Console.WriteLine(fun(20)); fun = target; Console.WriteLine(fun(5)); Func<string, int, string[]> fun2 = (s, i) => { return s.Split(new char[] { ' ' }, i); }; foreach (var m in fun2("this is a test", 4)) { Console.WriteLine(m); } } public void funTest3() { //action可以带参数,但没有返回值, Func<>必须有返回值 Action act1 = printf; act1(); Action<string> act2 = s => Console.WriteLine(s); act2("hellow world"); act2 = delegate(string s) { Console.WriteLine(s); }; act2("hello lxy"); } public void printf() { Console.WriteLine("this is a test"); } public bool target(int value) { return value > 10; } } }
Form1.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace delegateTest { public delegate string ConverFun(); public delegate string GetAString(); public delegate void ChangeUI(double num); public delegate void ChangeUIstr(string msg); public delegate void SortFun<T>(IList<T> sortary,Func<T,T,bool>compare); public partial class Form1 : Form { public Action<string> OutMessage; public Func<string, string> OutMessagw2; public Form1() { InitializeComponent(); btnTest1.Click += new EventHandler(btnTest1_Click); btnTest2.Click += new EventHandler(btnTest2_Click); btnTest3.Click += new EventHandler(btnTest3_Click); btnTest4.Click += new EventHandler(btnTest4_Click); btnTest5.Click += new EventHandler(btnTest5_Click); btnTest6.Click += new EventHandler(btnTest6_Click); btnTest7.Click += new EventHandler(btnTest7_Click); btnTest8.Click += new EventHandler(btnTest8_Click); btnTest9.Click += new EventHandler(btnTest9_Click); btnTest10.Click += new EventHandler(btnTest10_Click); btnTest11.Click += new EventHandler(btnTest11_Click); } void btnTest11_Click(object sender, EventArgs e) { test11(); } void btnTest10_Click(object sender, EventArgs e) { test10(); } void btnTest9_Click(object sender, EventArgs e) { test9(); } void btnTest8_Click(object sender, EventArgs e) { test8(); } void btnTest7_Click(object sender, EventArgs e) { test7(); } void btnTest6_Click(object sender, EventArgs e) { test6(); } void btnTest5_Click(object sender, EventArgs e) { test5(); } void btnTest4_Click(object sender, EventArgs e) { tes4(); } private void onChangeStr(string msg) { this.rtbMsgInfo.AppendText(string.Format("{0}\n",msg)); } private void onChage1(string msg) { this.rtbMsgInfo.AppendText(msg); } private string onChage2(string msg) { return msg; } private void onChange(double num) { this.rtbMsgInfo.AppendText(string.Format("{0}\n",num.ToString())); } void btnTest3_Click(object sender, EventArgs e) { test3(); } void btnTest2_Click(object sender, EventArgs e) { test2(); } void btnTest1_Click(object sender, EventArgs e) { test1(); } private void test11() { this.rtbMsgInfo.Clear(); rouEvent.CarDealer d1 = new rouEvent.CarDealer(); rouEvent.Consumer c1 = new rouEvent.Consumer("刘小勇"); rouEvent.Consumer c2 = new rouEvent.Consumer("姚军"); c1.onChange = onChangeStr; c2.onChange = onChangeStr; d1.NewCarEvent += c1.SubscriptionNewCarInfo; d1.NewCarEvent += c2.SubscriptionNewCarInfo; d1.PulishNewCarInfo("宝马1010"); MessageBox.Show("尝试编写弱事件, 无法引用这个类 WeekEventManager, 为什么?"); } private void test10() { this.rtbMsgInfo.Clear(); var dealer = new CarDealer(); var lxy = new Consumer("刘小勇"); dealer.onChange = onChangeStr; lxy.onChange = onChangeStr; dealer.NewCarInfo += lxy.NewCarIsHere; dealer.NewCar("宝马101"); var yj = new Consumer("姚军"); yj.onChange = onChangeStr; dealer.NewCarInfo += yj.NewCarIsHere; dealer.NewCar("宝马202"); dealer.NewCarInfo -= lxy.NewCarIsHere; dealer.NewCar("宝马303"); } private void test9() { this.rtbMsgInfo.Clear(); string str = "Lambda表达式:"; Func<string, string> lambda = param => { return str + param; }; this.rtbMsgInfo.AppendText(lambda("演示")+"\n"); //多个参数, 注意参数也可以显示指定变量类型 Func<double, double, string> lambda1 = (x, y) => { return (x * y).ToString(); }; this.rtbMsgInfo.AppendText(lambda1(3.0, 3.414)+"\n"); //只有一条语句可以不用return Func<double, double, double> lambda2 = (x, y) => x * y; this.rtbMsgInfo.AppendText(lambda2(4, 5).ToString()+"\n"); } private void test8() { //演示 匿名委托 //注意:匿名方法不能内部goto外部,或者外部goto内部. //也不能在匿名方法内使用不安全代码 this.rtbMsgInfo.Clear(); string s1 = "匿名委托"; Func<string, string> pro1 = delegate(string name) { return s1+name; }; this.rtbMsgInfo.AppendText(pro1(":演示")); } private void test7() { //演示 多播委托时,如果方法中有异常的处理办法 //通过调取委托列表,自己再循环遍历的办法,可以让一个方法异常后,不影响后面的方法继续遍历调用 this.rtbMsgInfo.Clear(); Class5 c5 = new Class5(); c5.onChange = onChange; c5.calfun(); } private void test6() { //演示 多播委托 this.rtbMsgInfo.Clear(); Class4 c4 = new Class4(); c4.onChange = onChange; c4.calfun(); } private void test5() { this.rtbMsgInfo.Clear(); Class3 c1 = new Class3(); int[] ary1 = new int[] { 33, 5, 11, 77, 66, 53, 18 }; c1.sort(ref ary1); //演示范型委托排序 Employee[] plist = new Employee[]{ new Employee("刘小勇",4100), new Employee("张辽",3800), new Employee("张飞",4000), new Employee("刘备",5200), new Employee("曹操",7200), new Employee("诸葛亮",2800), new Employee("蔡冒",1700) }; c1.sort1(plist, Employee.compare); foreach (Employee m in plist) { this.rtbMsgInfo.AppendText(string.Format("{0}\t{1}\n",m.Name,m.Salary)); } } private void tes4() { //演示 委托数组 this.rtbMsgInfo.Clear(); Class2 c1 = new Class2(); c1.onChange = onChange; c1.d3(); } private void test3() { //演示 隐式转换, 显示转换, 重载操作符 this.rtbMsgInfo.Clear(); myclass mc = 1;//通过隐式装换,生成myclass对象 rtbMsgInfo.AppendText(string.Format("{0}\n", mc.Value)); myclass mc2 = new myclass(2); rtbMsgInfo.AppendText(string.Format("{0}\n",(int)mc2));//显示转化,调用myclass至int的处理方法 rtbMsgInfo.AppendText(string.Format("{0}\n",mc2));//隐式转化,调用myclass至string的处理方法 } private void test2() { //演示 委托只验证签名与调用方法签名是否一致,并不关心是什么对象上调用该方法, //也不管是静态方法,还是实例方法. this.rtbMsgInfo.Clear(); int x = 40; GetAString firstStringMethod = x.ToString; rtbMsgInfo.AppendText(string.Format("String is {0}\n",firstStringMethod())); Currency balance = new Currency(34, 50); firstStringMethod = balance.ToString; rtbMsgInfo.AppendText(string.Format("String is {0}\n", firstStringMethod())); firstStringMethod = new GetAString(Currency.GetCurrencyUnit); rtbMsgInfo.AppendText(string.Format("String is {0}\n", firstStringMethod())); } private void test1() { //基本委托示例 this.rtbMsgInfo.Clear(); int k1 = 40; ConverFun fun = new ConverFun(k1.ToString); rtbMsgInfo.AppendText(string.Format("{0}\n",fun())); rtbMsgInfo.AppendText(string.Format("{0}\n",fun.Invoke())); } struct Currency { public uint Dollars; public ushort Cents; public Currency(uint dollars, ushort cents) { this.Dollars = dollars; this.Cents = cents; } public override string ToString() { return string.Format("${0}.{1,2:00}", Dollars, Cents); } public static string GetCurrencyUnit() { return "Dollar"; } public static explicit operator Currency(float value) { checked { uint dollars = (uint)value; ushort cents = (ushort)((value - dollars) * 100); return new Currency(dollars, cents); } } public static implicit operator float(Currency value) { return value.Dollars + (value.Cents / 100.0f); } public static implicit operator Currency(uint value) { return new Currency(value, 0); } public static implicit operator uint(Currency value) { return value.Dollars; } } private void btnTest12_Click(object sender, EventArgs e) { this.OutMessage = onChage1; onChage1("\nAction<>测试\n"); this.OutMessagw2 = onChage2; this.rtbMsgInfo.AppendText(OutMessagw2("\nFunc<>测试\n")); } private void button1_Click(object sender, EventArgs e) { var c8 = new Class8(); c8.OnException += new Class8.ExceptionDelegate(c8_OnException); c8.cal(2, 2); } void c8_OnException(string msg) { MessageBox.Show(msg); } private void button2_Click(object sender, EventArgs e) { new delegateTest().funTest(); new delegateTest().funTest2(); new delegateTest().funTest3(); } private void btnTest1_Click_1(object sender, EventArgs e) { } } }
运行结果:
40
40
Action<>测试
Func<>测试
String is 40
String is $34.50
String is Dollar
1
2
delegateTest.myclass
10.56
10.56
59.92
59.92
11.312
11.312
1.7424
1.7424
56.1001
56.1001
1.999396
1.999396
10.56
10.56
59.92
59.92
11.312
11.312
1.7424
1.7424
56.1001
56.1001
1.999396
1.999396
蔡冒1700
诸葛亮2800
张辽3800
张飞4000
刘小勇4100
刘备5200
曹操7200
25.12
9.8596
-999
9.8596
匿名委托:演示
Lambda表达式:演示
10.242
20
CarDealer, new car 宝马101
刘小勇: car 宝马101 is new
CarDealer, new car 宝马202
刘小勇: car 宝马202 is new
姚军: car 宝马202 is new
CarDealer, new car 宝马303
姚军: car 宝马303 is new
这个测试通不过
本站收费下载:
源码资料下载,扫码收费1元,勇哥用以支付本站服务器费用。
---------------------
作者:hackpig
来源:www.skcircle.com
版权声明:本文为博主原创文章,转载请附上博文链接!
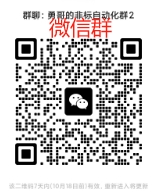
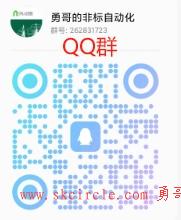