命名空间:sharClass.Settings
功能:保存界面控件内容到文件、读取配置文件内容到界面控件
功能详细说明:
Settings类可以保存常见控件内容到属性变量,你可以用它来保存设置面板的信息到磁盘文件,或者载入。
这时候控件的名字要加上前缀:txt, cbb,chk,以识别是TextBox、ComboBox、CheckBox
另外,这个类还可以保存PropertyGrid控件的信息。
如果像本程序那样申明了多个继承Settings类的对象时,要传递name以识别:
否则的话两个类会使用一个相同的名字进行变量存取。就看不到效果。
public myProperty(string name) : base(name) { }
常用函数列表:
public void UpdateFromDatabase() public void SetIntValue(string paraName, int value) public int? GetIntValue(string paraName) public void RemoveIntValue(string paraName) public void SetStringValue(string paraName, string value) public string GetStringValue(string paraName) public void RemoveStringValue(string paraName) public void SetBoolValue(string paraName, bool value) public bool? GetBoolValue(string paraName) public void RemoveBoolValue(string paraName) public void SetDoubleAryValue(string paraName,double[] value) public void SetDoubleValue(string paraName, double value) public double[] GetDoubleAryValue(string paraName) public double? GetDoubleValue(string paraName) public void RemoveDoubleValue(string paraName) public static bool SettingToProperty(Settings settings, string parentName, object obj, PropertyInfo pi) public static bool PropertyToSetting(Settings settings, string parentName, object obj, PropertyInfo pi) public static bool PropertyToControl(Dictionary<string, Control> ctrls, string parentName, object obj, PropertyInfo pi) public static bool PropertyToControl(Dictionary<string, Control> ctrls, string parentName, object obj, PropertyInfo pi, ref ToolTip Tip) public static string GetDecription(PropertyInfo pi) public static bool ControlToProperty(Dictionary<string, Control> ctrls, string parentName, object obj, PropertyInfo pi) public static Dictionary<string, Control> GetAllChildConfigControls(Control parent, string ControlType = null) /// 获得参数中的属性 public static List<PropertyInfo> GetConfigProperties(object obj) /// 将参数读到属性中 public static bool SettingsToProperties(Settings settings, object obj) /// 将属性设置到参数中 public static bool PropertiesToSettings(object obj, Settings settings) /// 将控件中参数读到属性中 public static bool ControlsToProperties(Control parentControl, object obj) /// 将参数属性设置到控件中 public static void ThPropertiesToControls(object obj, Control parentControl) /// 将属性设置到控件中 public static bool PropertiesToControls(object obj, Control parentControl) /// 将属性设置到控件中 public static void ThPropertiesToControls(object obj, Control parentControl, ref ToolTip tip) /// 将属性设置到控件中并且可以加ToolTip public static bool PropertiesToControls(object obj, Control parentControl, ToolTip tip) /// 属性设置到控件上 public static bool PropertiesToControls(object obj, Control parentControl, ref ToolTip tip) /// 将参数保存到默认的文件 public virtual bool Save() /// 保存参数到文件,文件路径可以设置 public virtual bool Save(string filePath) /// 加载参数 public virtual bool Load() /// 从路径加载参数 public virtual bool Load(string filePath)
参考例子:
这个例子演示了保存两个PropertyGrid控件和一组常规控件的内容到磁盘配置文件中去。
常规控件支持 TextBox, ComboBox, CheckBox
一般来说,PropertyGrid加上几个常规控件,足以应付配置面板的需要。
常规控件的命名必须为指定前缀+属性变量名。
TextBox, ComboBox, CheckBox的前缀依次为 txt, cbb, chk
下面的例子会让童鞋们体会到有了Settings类后,保存面板的设置项目会变得多么的容易。
using sharClass; using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace SettingsTest { public partial class Form1 : Form { appSetting set = new appSetting("myapp1"); myProperty myset1 = new myProperty("myapp2"); myProperty myset2 = new myProperty("myapp3"); private enum jobTypeEnum { 服务员,程序员,牛郎,铲屎官 } public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { cbbJob.DataSource= new enumHelper<jobTypeEnum>().getEnumItemArray(); pg1.SelectedObject = myset1; pg2.SelectedObject = myset2; loadConfig(); } private void saveConfig() { Settings.ControlsToProperties(this, set); myset1.Save(); myset2.Save(); set.Save(); } private void loadConfig() { Settings.PropertiesToControls(set, this); myset1.Load(); myset2.Load(); } private void btnSave_Click(object sender, EventArgs e) { saveConfig(); } } public partial class appSetting : Settings { [Config] public string Name1 { get; set; } [Config] public int Age { get; set; } [Config] public int WorkAge { get; set; } [Config] public string Job { get; set; } [Config] public bool loveMe { get; set; } public appSetting(string name) : base(name) { } } public class myProperty : Settings { [Config, Description("姓名"), Category("系统字段"), DefaultValue("")] public string UserName { get; set; } [Config, Description("年龄"), Category("系统字段"), DefaultValue(1)] public int UserAge { get; set; } [Config, Description("工年"), Category("系统字段"), DefaultValue(1)] public int UserWorkage { get; set; } public myProperty(string name) : base(name) { } /// <summary> /// 保存参数 /// </summary> /// <returns></returns> public override bool Save() { if (PropertiesToSettings(this, this)) return base.Save(); return false; } /// <summary> /// 加载参数 /// </summary> /// <returns></returns> public override bool Load() { if (base.Load()) return SettingsToProperties(this, this); return false; } } }
---------------------
作者:hackpig
来源:www.skcircle.com
版权声明:本文为博主原创文章,转载请附上博文链接!
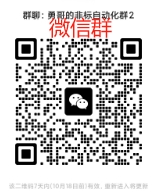
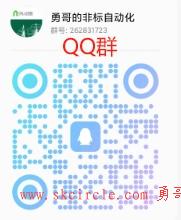