C# 外观模式(Facade)
http://www.skcircle.com/?id=1827
有关外观模式见下面贴子,下面是勇哥编写的一个例子。
FundClass.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace facadeTest1 { public class StockClass { private string _StockName; public string StockName { get { return _StockName; } set { _StockName = value; } } public StockClass(string stockname) { _StockName = stockname; } public string buy() { return string.Format("买入股票: [{0}] !", _StockName); } public string sell() { return string.Format("股票:[{0}] 卖出了!", _StockName); } } public class NationalDebtClass { private string _NationalDebtName; public string NationalDebtName { get { return _NationalDebtName; } set { _NationalDebtName = value; } } public NationalDebtClass(string nationaldebtName) { _NationalDebtName = nationaldebtName; } public string buy() { return string.Format("买入国债: [{0}] !", _NationalDebtName); } public string sell() { return string.Format("国债: [{0}] 卖出了!", _NationalDebtName); } } public class RealtyClass { private string _RealtyName; public string RealtyName { get { return _RealtyName; } set { _RealtyName = value; } } public RealtyClass(string realtryname) { _RealtyName = realtryname; } public string buy() { return string.Format("买入房地产: [{0}] !", _RealtyName); } public string sell() { return string.Format("产地产: [{0}] 卖出了!", _RealtyName); } } class FundClass { public ChangeUI onChange = null; public string msgInfo; StockClass stock1; StockClass stock2; NationalDebtClass nationalDebt1; RealtyClass realty1; public FundClass() { stock1 = new StockClass("新旺达新能源科技有限公司"); stock2=new StockClass("爱康电子科技有限公司"); nationalDebt1=new NationalDebtClass("中国人民银行国债"); realty1=new RealtyClass("武汉房地产"); } public void buy() { msgInfo = string.Format( stock1.buy()+Environment.NewLine+ stock2.buy()+Environment.NewLine+ nationalDebt1.buy()+Environment.NewLine+ realty1.buy()+Environment.NewLine ); if (onChange != null) onChange(); } public void sell() { msgInfo = string.Format( stock1.sell() + Environment.NewLine + stock2.sell() + Environment.NewLine + nationalDebt1.sell() + Environment.NewLine + realty1.sell() + Environment.NewLine ); if (onChange != null) onChange(); } } }
Delegate.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace facadeTest1 { public delegate void ChangeUI(); }
MVcontrol.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Windows.Forms; namespace facadeTest1 { class MVcontrol { private FundClass _fclass1; public MVcontrol(FundClass paramFundClass) { _fclass1 = paramFundClass; } public void UpdateUI(RichTextBox richControl) { richControl.Text = _fclass1.msgInfo; } } }
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace facadeTest1 { public partial class MainForm : Form { FundClass fund; MVcontrol mvc; public MainForm() { InitializeComponent(); fund = new FundClass(); fund.onChange = onChange; mvc = new MVcontrol(fund); btnBuy.Click += new EventHandler(btnBuy_Click); btnSell.Click += new EventHandler(btnSell_Click); } void btnSell_Click(object sender, EventArgs e) { fund.sell(); } void btnBuy_Click(object sender, EventArgs e) { fund.buy(); } public void onChange() { mvc.UpdateUI(this.richTextBox1); } private void btnSell_Click_1(object sender, EventArgs e) { } } }
本文出自勇哥的网站《少有人走的路》wwww.skcircle.com,转载请注明出处!讨论可扫码加群: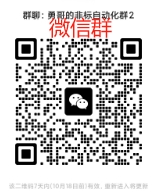
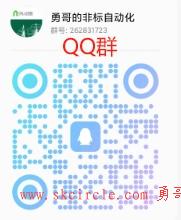
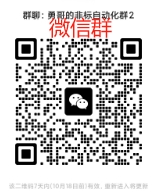
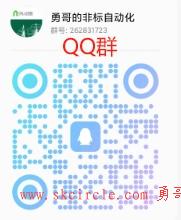