基础的二维变换是:平移,斜切,缩放,旋转,镜像
了解这个知识的目的是应用于像点胶设备中点位坐标的旋转上面。
源代码:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace yy { public partial class Form1 : Form { Graphics g; Point[] points = new Point[3]; Point[] pointa = new Point[3]; int n; public Form1() { InitializeComponent(); points[0] = new Point(30, 20); points[1] = new Point(200, 40); points[2] = new Point(200, 100); pointa = points; n = 3; } Point pingyi(Point b, int i, int j) //平移 { b.X += i; b.Y += j; return b; } Point suoxiao(Point a, double m) //放缩 { a.X = Convert.ToInt32(m * a.X); a.Y = Convert.ToInt32(m * a.Y); return a; } Point xuanzhuan(Point a, double c) //旋转 { int x = a.X; int y = a.Y; a.X = Convert.ToInt32(x*Math .Cos (c)-y *Math .Sin (c) ); a.Y = Convert.ToInt32(x*Math .Sin(c )+y *Math .Cos(c)); return a; } Point duichen(Point a,int i) //x轴对称 { if (i == 1) //x轴对称 { a.Y = -a.Y; } if (i == 2) //y轴对称 { a.X = -a.X; } if (i == 3) { a.X = -a.X; a.Y = -a.Y; } return a; } Point cuoqie(Point a, double b, double d) //错切 { int x=a.X; int y=a.Y; if (d == 0) { a.X =Convert .ToInt32( x + b * y); } else { a.Y = Convert .ToInt32( d * x + y); } return a; } void draw(Point[] pointm) //画图函数 { g = this.panel1.CreateGraphics(); g.Clear(panel1 . BackColor); g.TranslateTransform(215, 215); g.DrawLine(new Pen(new SolidBrush(Color.Black )), new Point(-300, 0), new Point(300, 0)); g.DrawLine(new Pen(new SolidBrush(Color.Black)), new Point(0, -300), new Point(0, 300)); g.DrawPolygon(new Pen(new SolidBrush(Color.Blue)), pointm); g.DrawPolygon(new Pen(new SolidBrush(Color.Blue)), pointm); g.Dispose(); } private void butret_Click(object sender, EventArgs e) //还原 { pointa[0] = new Point(30, 20); pointa[1] = new Point(200, 40); pointa[2] = new Point(200, 100); } private void butpingyi_Click(object sender, EventArgs e) //平移 { for (int i = 0; i < n; i++) { pointa[i] = pingyi(pointa[i], Convert.ToInt32(textBoxx.Text), Convert.ToInt32(textBoxy.Text)); } draw (pointa ); } private void butcuoqie_Click(object sender, EventArgs e) //错切 { double tana = Convert.ToDouble(textBoxjiaodu. Text); double a = Math.Tan(tana * Math.PI / 180); double b = 0, d = a; if (radioButton2.Checked == true) { d = 0; b = -a; } if (radioButton1.Checked == true) { d = 0; b = a; } if (radioButton3.Checked == true) { d = -a; b = 0; } if (radioButton4.Checked == true) { d = a; b = 0; } for (int i = 0; i < n; i++) { pointa[i] = cuoqie(pointa[i], b, d); } draw(pointa); } private void butsuofang_Click(object sender, EventArgs e) //缩放 { for (int i = 0; i < n; i++) { pointa[i] = pingyi(pointa[i], -pointa[0].X, -pointa[0].Y); //平移到原点 pointa[i] = suoxiao(pointa[i], Convert .ToDouble( textBoxsuo.Text) ); //放缩 pointa[i] = pingyi(pointa[i], pointa[0].X, pointa[0].Y); //平移回来 } draw(pointa); } private void button1_Click(object sender, EventArgs e) //画原始图形 { draw(pointa); } private void butxuanzhuan_Click(object sender, EventArgs e) { double c = Convert .ToInt32(textBoxzhuan.Text ) * 3.14 / 180; for (int i=0; i < n ; i++) { pointa[i] = xuanzhuan(pointa[i], c); //旋转 } draw(pointa); } private void butduichen_Click(object sender, EventArgs e) { int b = Convert.ToInt32(textBoxb.Text); double k = Convert.ToInt32(textBoxK.Text) ; g = this.panel1.CreateGraphics(); g.Clear(panel1.BackColor); g.TranslateTransform(215, 215); g.DrawLine(new Pen(new SolidBrush(Color.Black)), new Point(-300, 0), new Point(300, 0)); g.DrawLine(new Pen(new SolidBrush(Color.Black)), new Point(0, -300), new Point(0, 300)); g.DrawLine(new Pen(new SolidBrush(Color.DarkOrange)), new Point(0, b), new Point(60, Convert .ToInt32 ( 60*k+b))); g.DrawPolygon(new Pen(new SolidBrush(Color.Blue)), pointa); if (k == 90) { for (int i = 0; i < n; i++) { pointa[i] = duichen(pointa[i], 2); //关于y轴旋转 } } else { double c = Math.Atan(k); for (int i = 0; i < n; i++) { pointa[i] = pingyi(pointa[i], 0, -b); //平移到原点 pointa[i] = xuanzhuan(pointa[i], -c); //旋转 pointa[i] = duichen(pointa[i], 1); //关于x轴旋转 pointa[i] = xuanzhuan(pointa[i], c); //旋转 pointa[i] = pingyi(pointa[i], 0, b); //平移到原点 } } g.DrawPolygon(new Pen(new SolidBrush(Color.Blue)), pointa); g.Dispose(); } private void panel1_Paint(object sender, PaintEventArgs e) { } } }
---------------------
作者:hackpig
来源:www.skcircle.com
版权声明:本文为博主原创文章,转载请附上博文链接!
本文出自勇哥的网站《少有人走的路》wwww.skcircle.com,转载请注明出处!讨论可扫码加群: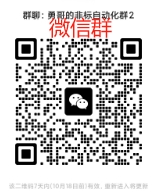
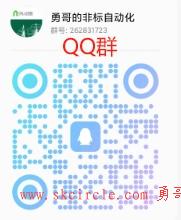
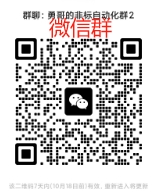
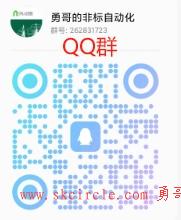