在工作流开发中,邮件通知是必不可少。这篇文章中,我将是使用WF4.0一步一步打造一个功能完整的邮件通知节点。
首先,新建一个WorkflowConsoleApplication项目,改名为MailNoticeDemo,如下图:
添加一个CodeActivity活动命名为MailNotice,添加一个ActivityDesigner活动命名为MailNoticeDesigner。项目结构如下图:
MailNotice用于写发送邮件的业务逻辑,MailNoticeDesigner用于设计活动的界面,现在MailNotice和MailNoticeDesigner是没有任何关联的,我们在MailNotice类上添加[Designer(typeof(MailNoticeDesigner))],关联MailNotice和MailNoticeDesigner,还需要引入System.ComponentModel命名空间,代码如下。
using System.Activities; using System.ComponentModel; using System.Activities.Presentation.Metadata; using System.Activities.Presentation.PropertyEditing; using System; namespace MailNoticeDemo { [Designer(typeof(MailNoticeDesigner))] public sealed class MailNotice : CodeActivity { ……… }
这时,MailNotice和MailNoticeDesigner活动中还没有进行编码。在项目中引入一个邮件发送类MailHelper.cs,编写MailNotice代码,如下:
[Designer(typeof(MailNoticeDesigner))] public sealed class MailNotice : CodeActivity { public InArgument<string> Receive { get; set; } public InArgument<string> Subject { get; set; } public InArgument<string> Content { get; set; } public string Attachment { get; set; } static MailNotice() { AttributeTableBuilder builder = new AttributeTableBuilder(); builder.AddCustomAttributes(typeof(MailNotice), "Attachment", new EditorAttribute(typeof(FilePickerEditor), typeof(DialogPropertyValueEditor))); MetadataStore.AddAttributeTable(builder.CreateTable()); } // If your activity returns a value, derive from CodeActivity<TResult> // and return the value from the Execute method. protected override void Execute(CodeActivityContext context) { SMTP smtp = new SMTP("你邮箱地址·", "显示的名称", new string[] { Receive.Get(context) }, null, null, Subject.Get(context), Content.Get(context), new string[] { Attachment }, "邮件发送服务", 25, "你邮箱地址·", "你邮箱密码", false); try { smtp.Send(); } catch (Exception ex) { string error = ex.Message; if (ex.InnerException != null) { error = ex.InnerException.Message; } Console.WriteLine("邮箱发送失败: " + error); } } }
设计MailNoticeDesigner活动的UI,设计UI之前让你可以先了解一下ExpressionTextBox。详见:expressiontextbox-101
通过expressiontextbox和WPF的控件,设计UI界面如下:
Xaml代码如下:
<sap:ActivityDesigner x:Class="MailNoticeDemo.MailNoticeDesigner" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:sap="clr-namespace:System.Activities.Presentation;assembly=System.Activities.Presentation" xmlns:sapc="clr-namespace:System.Activities.Presentation.Converters;assembly=System.Activities.Presentation" xmlns:sapv="clr-namespace:System.Activities.Presentation.View;assembly=System.Activities.Presentation" mc:Ignorable="d" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" d:DesignHeight="221" d:DesignWidth="336"> <sap:ActivityDesigner.Resources> <ResourceDictionary x:Uid="ResourceDictionary_1"> <sapc:ArgumentToExpressionConverter x:Uid="sadv:ArgumentToExpressionConverter_1" x:Key="ArgumentToExpressionConverter" /> </ResourceDictionary> </sap:ActivityDesigner.Resources> <Grid Height="190" Width="328"> <Grid.RowDefinitions> <RowDefinition Height="28"></RowDefinition> <RowDefinition Height="28"></RowDefinition> <RowDefinition Height="54"></RowDefinition> <RowDefinition Height="47" /> <RowDefinition Height="31*" /> </Grid.RowDefinitions> <Grid.ColumnDefinitions> <ColumnDefinition Width=".2*"></ColumnDefinition> <ColumnDefinition Width=".8*"></ColumnDefinition> </Grid.ColumnDefinitions> <Label Content="收件人" Height="28" HorizontalAlignment="Left" Grid.Row="0" Grid.Column="0" Name="label1" VerticalAlignment="Top" /> <sapv:ExpressionTextBox Grid.Row="0" Grid.Column="1" OwnerActivity="{Binding Path=ModelItem}" Expression="{Binding Path=ModelItem.Receive, Mode=TwoWay, Converter={StaticResource ArgumentToExpressionConverter}, ConverterParameter=In }" UseLocationExpression="False" /> <Label Content="主题:" Grid.Row="1" Grid.Column="0" HorizontalAlignment="Left" Name="label2" VerticalAlignment="Top" /> <sapv:ExpressionTextBox Grid.Row="1" Grid.Column="1" OwnerActivity="{Binding Path=ModelItem}" Expression="{Binding Path=ModelItem.Subject, Mode=TwoWay, Converter={StaticResource ArgumentToExpressionConverter}, ConverterParameter=In }" UseLocationExpression="False" /> <Label Content="正文:" Grid.Row="2" HorizontalAlignment="Left" Name="label3" VerticalAlignment="Top" /> <sapv:ExpressionTextBox Grid.Row="2" Grid.Column="1" OwnerActivity="{Binding Path=ModelItem}" Expression="{Binding Path=ModelItem.Content, Mode=TwoWay, Converter={StaticResource ArgumentToExpressionConverter}, ConverterParameter=In }" UseLocationExpression="False" Margin="0,0,0,1" Grid.RowSpan="2" /> </Grid> </sap:ActivityDesigner>
样我们就完成了这个邮件活动。测试一下。在Workflow1中拖入这个活动。输入收件人、主题、正文,如下图:
设置附件,如下图:
启动工作流发送邮件:
WorkflowInvoker.Invoke(new Workflow1());
你会发现这个活动的图标不够美观,让我们修改一下这个自定义活动的图标。 在MailNoticeDesigner.xaml中加入下面代码
<sap:ActivityDesigner.Icon> <DrawingBrush> <DrawingBrush.Drawing> <ImageDrawing> <ImageDrawing.Rect> <Rect Location="0,0" Size="16,16" ></Rect> </ImageDrawing.Rect> <ImageDrawing.ImageSource> <BitmapImage UriSource="mail.ico" ></BitmapImage> </ImageDrawing.ImageSource> </ImageDrawing> </DrawingBrush.Drawing> </DrawingBrush> </sap:ActivityDesigner.Icon>
设置图标mail.ico,将Build action设置为Resource,如下图:
看一下我们最终打造的邮件活动:
总结:这篇文章详细讲解了创建WF4.0一个自定义活动的完整过程,虽然很简单,但对创建自定义活动很有参考价值。
代码:
/Files/zhuqil/MailNoticeDemo.rar
转载自:
https://www.cnblogs.com/zhuqil/archive/2010/04/29/wfmail.html
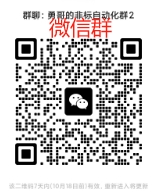
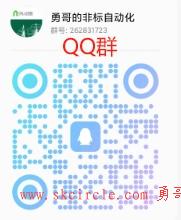